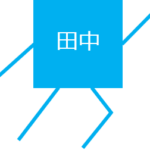
田中太郎
連想配列(associative array)についてまとめます
連想配列のmethod
<key_type>は連想配列のキーの型です
method | 内容 | 引数 | 戻り値 |
---|---|---|---|
a.num() | aの要素数を返す | なし | int |
a.exists(<key>) | aの要素に<key>があれば1、それ以外は0を返す | <key_type> | <key_type> |
a.delete(<key>) | aの要素から<key>を削除する | <key_type> | なし |
a.first(<変数>) | aの要素の先頭(0→9, a→z)を<変数>に代入する | ref <key_type> | int |
a.last(<変数>) | aの要素の最後(0→9, a→z)を<変数>に代入する | ref <key_type> | int |
a.next(<変数>) | aの<変数>の次の要素を<変数>に代入する | ref <key_type> | int |
a.prev(<変数>) | aの<変数>の前の要素を<変数>に代入する | ref <key_type> | int |
サンプルコード
a.num()
module tb;
int a[string];
initial begin
a["alpha"] = 1;
a["beta"] = 2;
a["charlie"] = 3;
$display("%d", a.num());
$finish;
end
endmodule
// 出力
// 3
a.exists()
module tb;
int a[string];
initial begin
a["alpha"] = 1;
a["beta"] = 2;
a["charlie"] = 3;
$display("%d", a.exists("delta")); // 存在しないから0
$display("%d", a.exists("alpha")); // 存在するから1
$finish;
end
endmodule
// 出力
// 0
// 1
a.delete()
module tb;
int a[string];
initial begin
a["alpha"] = 1;
a["beta"] = 2;
a["charlie"] = 3;
a.delete("alpha");
$display("%d", a.exists("alpha")); // 存在しないから0
$finish;
end
endmodule
// 出力
// 0
a.first()
module tb;
int a[string];
int b;
string c;
initial begin
a["alpha"] = 1;
a["beta"] = 2;
a["charlie"] = 3;
b = a.first(c);
$display("%s", c);
$finish;
end
endmodule
// 出力
// alpha
a.last()
module tb;
int a[string];
int b;
string c;
initial begin
a["alpha"] = 1;
a["beta"] = 2;
a["charlie"] = 3;
b = a.last(c);
$display("%s", c);
$finish;
end
endmodule
// 出力
// charlie
a.next()
module tb;
int a[string];
int b;
string c;
initial begin
a["alpha"] = 1;
a["beta"] = 2;
a["charlie"] = 3;
c = "beta";
b = a.next(c);
$display("%s", c);
$finish;
end
endmodule
// 出力
// charlie
a.prev()
module tb;
int a[string];
int b;
string c;
initial begin
a["alpha"] = 1;
a["beta"] = 2;
a["charlie"] = 3;
c = "beta";
b = a.prev(c);
$display("%s", c);
$finish;
end
endmodule
// 出力
// alpha
まとめ
連想配列についてまとめました
コメント