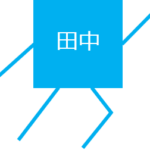
田中太郎
stringのmethod(組み込みfunction/task) についてまとめます
string型のmethod
method | 内容 | 引数 | 戻り値 |
---|---|---|---|
a.len() | aの長さを返す | なし | int |
a.putc(<位置>, <文字>) | aの<位置>と<文字>を置換する | int,byte | なし |
a.getc(<位置>) | aの<位置>の文字を返す | int | string |
a.toupper() | aをすべて大文字にする | string | string |
a.tolower() | aをすべて小文字にする | string | string |
a.compare(<文字列>) | aと<文字列>が同じとき0を返す | string | int |
a.icompare(<文字列>) | aと<文字列>が同じとき0を返す (大文字小文字を区別しない) | string | int |
a.substr(<開始>, <終了>) | aの<開始>から<終了>までの文字列を返す | int, int | string |
a.atoi() | aを10進数に変換する | なし | int |
a.atohex() | aを16進数に変換する | なし | int |
a.atooct() | aを8進数に変換する | なし | int |
a.atobin() | aを2進数に変換する | なし | int |
a.atoreal() | aを実数に変換する | なし | real |
a.itoa(<10進数>) | <10進数>を文字列型に変換する | int | なし |
a.hextoa(<16進数>) | <16進数>を文字列型に変換する | int | なし |
a.octtoa(<8進数>) | <8進数>を文字列型に変換する | int | なし |
a.bintoa(<2進数>) | <2進数>を文字列型に変換する | int | なし |
a.realtoa(<実数>) | <実数>を文字列型に変換する | real | なし |
サンプルコード
a.len()
module tb;
string a = "abcde";
int b;
initial begin
b = a.len();
$display("%d", b);
$finish;
end
endmodule
// 出力
// 5
a.putc()
module tb;
string a = "abcde";
initial begin
a.putc(0, "N");
$display("%s", a);
$finish;
end
endmodule
// 出力
// Nbcde
a.getc()
module tb;
string a = "abcde";
int b;
initial begin
b = a.getc(0);
$display("%s", b);
$finish;
end
endmodule
// 出力
// a
a.toupper()
module tb;
string a = "abcde";
string b;
initial begin
b = a.toupper();
$display("%s", b);
$finish;
end
endmodule
// 出力
// ABCDE
a.tolower()
module tb;
string a = "ABCDE";
string b;
initial begin
b = a.tolower();
$display("%s", b);
$finish;
end
endmodule
// 出力
// abced
a.compare()
module tb;
string a = "abcde";
int b;
initial begin
b = a.compare("abcde");
$display("%d", b);
b = a.compare("abc");
$display("%d", b);
$finish;
end
endmodule
// 出力
// 0
// 2
a.icompare()
module tb;
string a = "abcde";
int b;
initial begin
b = a.compare("ABCDE"); // compareは大文字小文字を区別する
$display("%d", b);
b = a.icompare("ABCDE"); // icompareは大文字小文字を区別しない
$display("%d", b);
$finish;
end
endmodule
// 出力
// 1
// 0
a.substr()
module tb;
string a = "abcde";
string b;
initial begin
b = a.substr(1, 3);
$display("%s", b);
$finish;
end
endmodule
// 出力
// bcd
a.atoi(), a.atohex(), a.atooct(), a.atobin(), a.atoreal()
module tb;
string a = "100";
string b = "3.14";
initial begin
$display("%d", a.atoi());
$display("%d", a.atohex());
$display("%d", a.atooct());
$display("%d", a.atobin());
$display("%.2f", b.atoreal());
$finish;
end
endmodule
// 出力
// 100
// 256
// 64
// 4
// 3.14
a.itoa(), a.hextoa(), a.octtoa(), a.bintoa(), a.realtoa()
module tb;
string a;
initial begin
a.itoa(100);
$display("%s", a);
a.hextoa(100);
$display("%s", a);
a.octtoa(100);
$display("%s", a);
a.bintoa(100);
$display("%s", a);
a.realtoa(3.14);
$display("%s", a);
$finish;
end
endmodule
// 出力
// 100
// 64
// 144
// 1100100
// 3.14
まとめ
string型のメソッドについてまとめました
コメント