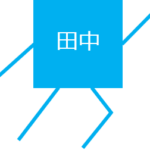
田中太郎
SystemVerilogのサンプルコード集です
Dフリップフロップ
module dff #(
parameter BIT_WIDTH = 9
)(
input clk,
input rst_n,
input [BIT_WIDTH-1:0] in_data,
output logic [BIT_WIDTH-1:0] out_data
);
always_ff @(posedge clk, negedge rst_n) begin
if (!rst_n) begin
out_data <= {BIT_WIDTH{1'b0}};
end
else begin
out_data <= in_data;
end
end
endmodule
Dフリップフロップ (Enable付き)
module dff_en #(
parameter BIT_WIDTH = 9
)(
input clk,
input rst_n,
input en,
input [BIT_WIDTH-1:0] in_data,
output logic [BIT_WIDTH-1:0] out_data
);
always_ff @(posedge clk, negedge rst_n) begin
if (!rst_n) begin
out_data <= {BIT_WIDTH{1'b0}};
end
else begin
if (en) begin
out_data <= in_data;
end
end
end
endmodule
カウンタ
module counter #(
parameter BIT_WIDTH = 9
)(
input clk,
input rst_n,
input en,
output logic [BIT_WIDTH-1:0] data
);
always_ff @(posedge clk, negedge rst_n) begin
if (!rst_n) begin
data <= {BIT_WIDTH{1'b0}};
end
else begin
if (en) begin
data <= data + 1'b1;
end
end
end
endmodule
クリップ
module clip #(
parameter BW_INPUT = 3,
parameter BW_VALID = 2
)(
input clk,
input rst_n,
input [BW_INPUT-1:0] din,
output logic [BW_VALID-1:0] dout
);
always_ff @(posedge clk, negedge rst_n) begin
if (!rst_n) begin
dout <= {BW_VALID{1'b0}};
end
else begin
if (din[BW_INPUT-1:BW_VALID] == {BW_INPUT-BW_VALID{1'b0}}) begin
dout <= din[BW_VALID-1:0];
end
else begin
dout <= {BW_VALID{1'b1}};
end
end
end
endmodule
加算後クリップ
module clip_add #(
parameter BW_INPUT = 3,
parameter BW_VALID = 2
)(
input clk,
input rst_n,
input [BW_INPUT-1:0] in0,
input [BW_INPUT-1:0] in1,
output logic [BW_VALID-1:0] data
);
logic [BW_INPUT:0] tmp;
assign tmp = in0 + in1;
always_ff @(posedge clk, negedge rst_n) begin
if (!rst_n) begin
data <= {BW_VALID{1'b0}};
end
else begin
if (tmp[BW_INPUT:BW_VALID] == {BW_INPUT+1-BW_VALID{1'b0}}) begin
data <= tmp[BW_VALID-1:0];
end
else begin
data <= {BW_VALID{1'b1}};
end
end
end
endmodule
減算後クリップ
module clip_subtraction #(
parameter BW_INPUT = 3,
parameter BW_VALID = 3
)(
input clk,
input rst_n,
input [BW_INPUT-1:0] in0,
input [BW_INPUT-1:0] in1,
output logic [BW_VALID-1:0] data
);
logic [BW_INPUT:0] tmp;
assign tmp = in0 - in1;
always_ff @(posedge clk, negedge rst_n) begin
if (!rst_n) begin
data <= {BW_VALID{1'b0}};
end
else begin
if (tmp[BW_INPUT:BW_VALID] == {BW_INPUT+1-BW_VALID{1'b0}}) begin
data <= tmp[BW_VALID-1:0];
end
else begin
data <= {BW_VALID{1'b0}};
end
end
end
endmodule
乗算回路
module mult #(
parameter BIT_WIDTH_0 = 3,
parameter BIT_WIDTH_1 = 3
)(
input clk,
input rst_n,
input [BIT_WIDTH_0-1:0] in0,
input [BIT_WIDTH_1-1:0] in1,
output logic [BIT_WIDTH_0+BIT_WIDTH_1-1:0] data
);
always_ff @(posedge clk, negedge rst_n) begin
if (!rst_n) begin
data <= {BIT_WIDTH_0+BIT_WIDTH_1{1'b0}};
end
else begin
data <= in0 * in1;
end
end
endmodul
シフト演算
module shift #(
parameter BW_IN = 3
)(
input clk,
input rst_n,
input [BW_IN-1:0] in0,
input [1:0] shift_num,
output logic [BW_IN+3-1:0] data
);
always_ff @(posedge clk, negedge rst_n) begin
if (!rst_n) begin
data <= {BW_IN+3{1'b0}};
end
else begin
case(shift_num)
2'b00 : data <= in0 << 0;
2'b01 : data <= in0 << 1;
2'b10 : data <= in0 << 2;
default: data <= in0 << 3;
endcase
end
end
endmodule
昇順並び替え(2入力)
module sort2 #(
parameter BIT_WIDTH = 9
)(
input clk,
input rst_n,
input [BIT_WIDTH-1:0] in0,
input [BIT_WIDTH-1:0] in1,
output logic [BIT_WIDTH-1:0] sorted0,
output logic [BIT_WIDTH-1:0] sorted1
);
always_ff @(posedge clk, negedge rst_n) begin
if (!rst_n) begin
sorted0 = {BIT_WIDTH{1'b0}};
sorted1 = {BIT_WIDTH{1'b0}};
end
else begin
if (in0 > in1) begin
sorted0 = in0;
sorted1 = in1;
end
else begin
sorted0 = in1;
sorted1 = in0;
end
end
end
endmodule
インスタンス
module top (
input clk,
input rst_n,
output logic dout
);
sub #(
) u_sub(
.clk(clk),
.rst_n(rst_n),
.dout(dout)
);
endmodule
module sub (
input clk,
input rst_n,
output logic dout
);
always_ff @(posedge clk, negedge rst_n) begin
if (!rst_n) begin
dout <= 1'b0;
end
else begin
dout <= 1'b1;
end
end
endmodule
コメント